In this post, I have an Interactive Report which I have added a checkbox for each row. I tried to keep the state of each checkbox (checked or unchecked) even if I transfer between pages of the Interactive Report or reload the page. So I have used the "Local Storage" of the Browser as a place to maintain and retrieve the checked rows.
1- Create an Interactive Report region with below code and set Static ID to “MyReport”:
select EMPNO,
ENAME,
JOB,
DEPTNO,
apex_item.checkbox2(
p_idx => 1,
p_value => empno,
p_attributes => 'class="selected-chkbox"'
) checkbox
from EMP
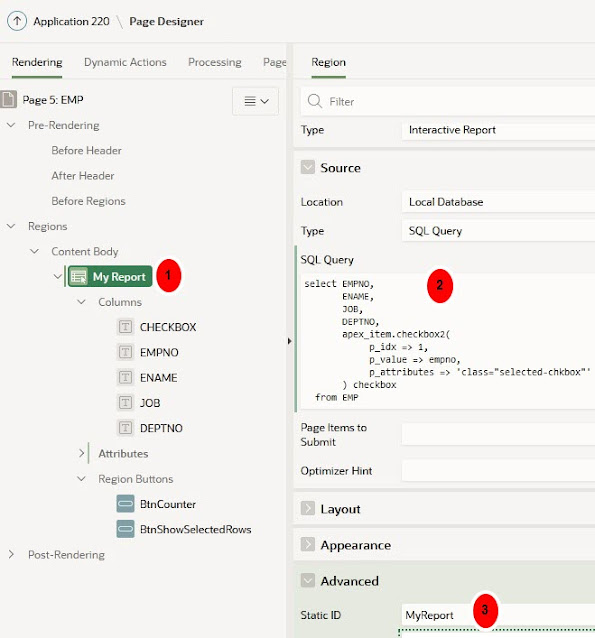
* Set “Heading” of the “chekbox field” to <input type="checkbox" id="ChkSelectAll"> All
*Disable all of features in “Enable Users To” section of the “chekbox field”.
* Disable “Escape special characters” of the “chekbox field”.
2- Add a button of type “Defined by Dynamic Action” and set Static ID to “BtnCounter” .
This button is used to display the number of selected rows as well as uncheck them.
3- Add a button of type “Defined by Dynamic Action” and set Static ID to “BtnShowSelectedRows” .
This button is used to display the selected rows.
4- Add below JavaScript in “Function and Global Variable Declaration” of page :
var myStorageName = "v_checked_list";
/**
* return json object of the local storage value
*/
function getLocalStorageJson() {
var strItems = localStorage.getItem(myStorageName);
var jItems = [];
if(strItems != null && strItems!="") {
jItems = JSON.parse(strItems);
}
return jItems;
}
/**
* set the local storage
* @param {object} jItems json value to set
*/
function setLocalStorage(jItems) {
localStorage.setItem(myStorageName, JSON.stringify(jItems));
}
/**
* clear the local storage
*/
function clearLocalStorage() {
localStorage.setItem(myStorageName, '');
$('.selected-chkbox,#ChkSelectAll').prop('checked', false);
$('#BtnCounter').html('Selected Rows: 0');
}
/**
* add/remove row value from local storage
* @param {object} $obj jquery checkbox object
*/
function setStorageByCheck($obj) {
var jItems = getLocalStorageJson();
var idof = $obj.val();
var found = jItems.some(el => parseInt(el.id) == parseInt(idof));
if ($obj.is(':checked')) {
if(!found) {
jItems.push({id:idof});
}
}
else {
if(found) {
jItems = jItems.filter(el => parseInt(el.id) != parseInt(idof));
}
}
setLocalStorage(jItems);
var lsCount = getLocalStorageJson().length;
$('#BtnCounter').html('Selected Rows: ' + lsCount);
}
/**
* clear all checked rows and local storage
*/
function clearAllCheckedRow() {
if(getLocalStorageJson().length==0)
return;
apex.message.confirm('are you sure to uncheck all the selected rows?', function(ok) {
if(ok) {
clearLocalStorage();
}
});
}
/**
* set check for rows, according to the local storage
*/
function applyCheckForRows() {
var jItems = getLocalStorageJson();
if(jItems.length > 0) {
$.each(jItems, function(key,val) {
$('.selected-chkbox[value="'+val.id+'"]').prop('checked',true);
})
}
else {
$('.selected-chkbox').prop('checked', false);
}
}
/**
* select all rows
*/
function selectAll() {
var getItems = [];
$('.selected-chkbox').each(function() {
$(this).prop('checked',true);
setStorageByCheck($(this));
});
}
/**
* unselect all rows
*/
function unSelectAll() {
$('.selected-chkbox').each(function() {
$(this).prop('checked',false);
setStorageByCheck($(this));
});
}
function showLocalStorageData() {
var sret = [];
var jItems = getLocalStorageJson();
$.each(jItems, function(key,val) {
sret.push(val.id);
})
alert(sret.toString().replace(/,/gi,', '));
}
* Then add below javascript code in “Execute when Page Loads” :
var removeOnLoad = false; //--> this flag can be set to `true` or `false`
if(removeOnLoad)
clearLocalStorage();
else {
applyCheckForRows();
$('#BtnCounter').html('Selected Rows: ' + getLocalStorageJson().length);
}
$(document).on('change','#ChkSelectAll',function() {
if($(this).is(':checked'))
selectAll();
else
unSelectAll();
});
$(document).on('change','.selected-chkbox',function() {
setStorageByCheck($(this));
});
$('#BtnCounter').click(function() {
clearAllCheckedRow();
});
$('#BtnShowSelectedRows').click(function() {
showLocalStorageData();
});
$("#MyReport").on("apexafterrefresh", function(e) {
applyCheckForRows();
});
As you can see, there is a variable called "v_checked_lists" in the browser storage to hold the selected rows.
I hope you enjoyed reading this blog post.
Nice Post and intelligent idea. Great!
ReplyDelete